How to create arrays in PHP?
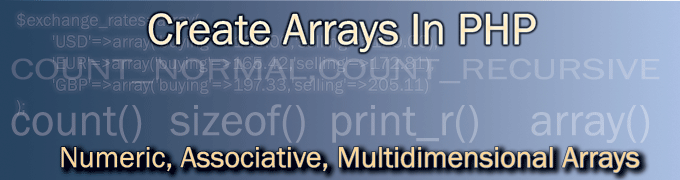
What is an array in php?
An Array is a collection of data values. It can also define as a collection of key/value pairs. An array can store multiple values in as a single variable. because array store values under a single variable name. Each array element has index which is used to access the array element. An array can also store diffrent type of data values. That means you can store integer and string values in the same array. PHP has lot of array functions to deal with arrays. You can store integers, strings, floats or objects in arrays. Arrays are very important in any programming language. You can get complete idea of arrays by reading this tutorial.
How to define an array in PHP?
$numbers = array(1,2,3,4,5,6,7,8,9,0);This will create an array of 10 elements. array is the pHP keyword that use to define a array. Not like other programming languages array size is not a fixed value. You can expand it at any time. That means you can store any value in the array you create. PHP will ready to adjust the size of the array.
Why arrays are important?
- Store similar data in a structured manner.
- Easy to access and change values.
- You can define any number of values (No need to define multiple variables).
- Save time and resources.
- To store temporary data.
In PHP there are two types of arrays.
- Numeric/Indexed Arrays
- Associative Arrays
Numeric Arrays
An indexed or a numeric array stores its all elements using numerical indexes. Normally indexed array begins with 0 index. When adding elements to the array index will be automatically increased by one. So always maximum index may be less than one to the size of the array. Following example shows you how to declare numeric arrays in pHPe.g. $programming_languages = array('PHP','Perl','C#');
Also you can define indexed/numeric arrays as follows.
$programming_languages[0]='PHP';
$programming_languages[1]='Perl';
$programming_languages[2]='C#';
Actually you don't need to specify the index, it will be automatically created. So you can also define it as bellow.
$programming_languages[]='PHP';
$programming_languages[]='Perl';
$programming_languages[]='C#';
Above array has three elements. The value 'PHP' has the index of 0 while 'C#' has index of 2. You can add new elements to this array. Then index will be increased one by one. That means the next element index will be 3. You can use that integer index to access array index as follows
echo $programming_languages[2];//This will be print 'C#'
or
echo $programming_languages{2}; //You can also use curly brackets
If you access an element which is not exists in the numeric array you will get an error message like Notice: Undefined offset: 4 in D:\Projects\array.php on line 7.
Associative Arrays
Associative arrays have keys and values. It is similar to two-column table. In associative arrays, key is use to access the its value. Every key in an associative array unique to that array. That means you can't have more than one value with the same key. Associative array is human readable than the indexed arrays. Because you can define a key for the specific element. You can use single or double quotes to define the array key. Following example explains you how to declare associative arrays in pHP
$user_info = array('name'=>'Nimal','age'=>20,'gender'=>'Male','status'=>'single');
Method 2:
$user_info['name']='Nimal';
$user_info['age']='20';
$user_info['gender']='Male';
$user_info['status']='Single'; Above array has four key pair values. That means array has 4 elements. Each element has a specific key. We use that key to access array element in associative arrays. You can't duplicate the array key. If there a duplicate key, the last element will be used.
echo $user_info['name'];//This will print Nimal
or
echo $user_info{'name'};
If you access an element which is not exists in the associative array you will get an error message like Notice: Undefined index: country in D:\Projects\array.php on line 11.
Multidimensional Arrays
Multidimensional arrays are arrays of arrays. That means one element of the array contains another array. Like wise you can define very complex arrays in pHP Suppose you have to store marks of the students according to their subjects in a class room using an array, You can use a multidimensional array to perform the task. See bellow example.Syntax:
$marks=array(
'nimal'=>array('English'=>67,'Science'=>80,'Maths'=>70),
'sanka'=>array('English'=>74,'Science'=>56,'Maths'=>60),
'dinesh'=>array('English'=>46,'Science'=>65,'Maths'=>52)
);
echo $marks['sanka']['English'];//This will print Sanka's marks for English
You can use array_keys() method to get all keys of an array. This will returns an array of keys.
print_r(array_keys($marks));
//This will output Array ( [0] => nimal [1] => sanka [2] => dinesh )
Note: you can use student number and subject number instead of using student name and subject name.
1. How to loop/iterate through an array in pHP?
if there are large number of elements in an array, It is not practical to access elements by manually. So you have to use loops for it. You can use foreach and for loops for access array elements. I think foreach loop is the most convenient method to access array elements. because you don't need to know the size of the array. In this tutorial you can learn iterate through arrays.
<?pHP
//Using foreach loop
$days = array('Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday');
foreach($days as $day){
echo $day.'<br/>';
}
//Using for loop
$programmingLanguages = array('PHP','Python','Perl','Java','C#','VB.Net','C++');
for($x=0;$x<count($programmingLanguages);$x++){
echo $programmingLanguages[$x].'<br/>';
}
//Also you can print all array elements using print_r() method. This is useful to determine array structure.
print_r($countries);
//This will out put Array ( [0] => SriLanka [1] => India [2] => America [3] => UK [4] => Japan [5] => China )
? >
2. How to get the size of an array using pHP?
Sometimes you may need to get how many elements exists in the array or the size of the array. You can use pHP in-built methods to find the size of a any array. See bellow examples. Also you can count all elements in a multidimensional array using count() function.
<?pHP
$countries = array('SriLanka','India','America','UK','Japan','China');
echo count($countries); //using count() method
echo '<br/>';
echo sizeof($countries);// using sizeof() method
//Get multidimensional array size
$exchange_rates=array(
'USD'=>array('buying'=>125.00,'selling'=>129.00),
'EUR'=>array('buying'=>165.42,'selling'=>172.81),
'GBP'=>array('buying'=>197.33,'selling'=>205.11)
);
echo count($exchange_rates,true);// this will out put 9
//or
echo count($exchange_rates,COUNT_RECURSIVE);
echo count($exchange_rates,COUNT_NORMAL);// this will out put 3
?>
2 comments:
Hi Guys,
Looking for some desperately.
I am new to php, with a lot search I have been able to put together some form of working script. The problem I am now facing is I have multiple checkboxes for the users to select from but the selected checkbox value does not get inserted
into the table instead shows as “Array”. With some help I plan to get all the selected checkbox values into one table.
I have two files one "testpage.html" which contains the form and the other "test_post.php" to process the form data. Please find below html fields and php file codes.
Really appreciate your help in advance.
HTML form fields are:
Full Name: (type=text)
Number: (type=text)
Email Address: (type=text)
Address: (type=text)
Which animal do you want to keep? Dog(type=checkbox)
Cat (type=checkbox)
Lion (type=checkbox)
Tiger (type=checkbox)
Leopard (type=checkbox)
submit (value=submit)
PHP code:
";
echo "Back to main page";
}
else {
echo "ERROR";
}
?>
It’s a really very informative information. It’s very useful and knowledgeable for me. I will bookmark this page and come soon to see the updates.
Post a Comment