How to create compressed/zip file using PHP?
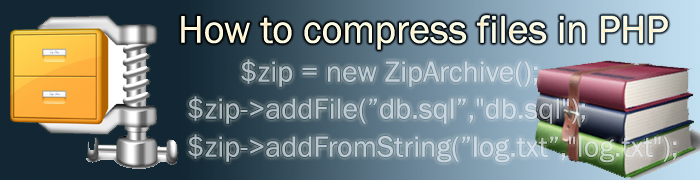
What is a compressed/zip file?
A zip file is a file that contains one or more compressed files in it. That means the size of the compressed file is lower than the original file(s) and can hold many files inside it. .zip file extension is use to identify compressed files.
There are number of compressed file formats. .zip, .rar, .7z and .gz are well known formats among them. compressed or archived files are useful when backup your data. Because if consumes less space and easily copy into removable media such as pen drive. Suppose that you have to develop a web application that sends some files to the client attached with a mail. In such case instead of attaching every file to the e-mail, you can create a compressed zip file and attach that file to the mail. Also when you are creating SQL backups, you can compressed those SQL files to save disk space and server bandwidth.
Create ZIP compressed archive files using PHP?
PHP has in-built support for creating and reading compressed archived files. In latest PHP releases (PHP 5.3 or higher) Zip extension is included by default. But in previous releases you have to enable php_zip.dll in php.ini file. PHP use ZipArchive class to provide zip functionality. It is very easy to use. Using this extension you can create and read zip/compressed files. Also you can create zip files from string inputs or by using files. This tutorial explain everything that you need to know when creating compressed files in PHP.
How to check if zip extension is loaded?
You can make sure Zip extension is loaded as follows. To check whether an extension is loaded or not you can use extension_loaded function.
<?php
if(extension_loaded('zip')){
echo 'PHP zip extension is loaded';
}else{
echo 'PHP zip extension is not loaded';
}
?>
Also you can use phpinfo(INFO_MODULES) to check if the module is loaded. check zip extension is enabled.
1. How to create zip file using string input in PHP?
Following example code shows you to create a compressed archive file using string. That means you can make a zip file with a string input. In this exampl, entered text is convert to a text file and then it convert to a zip file. First of all check the demo program.
<?php
$zip = new ZipArchive();
$archive_name = "myZip.zip";
if ($zip->open($archive_name, ZIPARCHIVE::CREATE)!==TRUE) {
exit("Error while opening".$archive_name);
}
$zip->addFromString("testfile.txt", "You can create zip files from strings. here is the example tutorial.\n");
$zip->close();
echo 'File size = '.number_format((filesize($archive_name)/1024),2).' Kb';
?>
Above code will generate a zip file called 'myZip.zip' file in the directory which you run the PHP script. Make sure you have permission to create files in the folder. Also you can get the size of the created zip file.
Note: You can add more than one string to the zip file. You can add multiple strings as separate files in to the zip file. Checkout bellow example to add multiple files to the archive.
<?php
$zip = new ZipArchive();
$archive_name = "multiple.zip";
if ($zip->open($archive_name, ZIPARCHIVE::OVERWRITE)!==TRUE) {
exit("Error while opening $archive_name");
}
$zip->addFromString("first.txt", "This is the first string input.\n");
$zip->addFromString("second.txt", "This is the second string input.\n");
$zip->addFromString("third.txt", "This is the third string input. Likewise you can add multiple strings to create zip file\n");
$zip->close();
echo 'File size = '.number_format((filesize($archive_name)/1024),2).' Kb';
?>