How to read image Metadata using PHP?
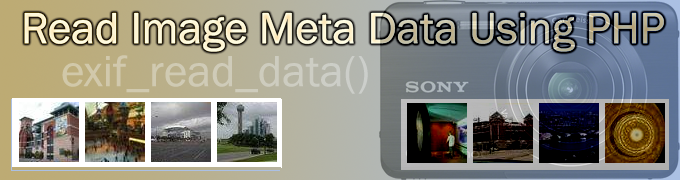
What is image Metadata?
Image Metadata refers to piece of information that stored in a photo. These information are automatically embedded to the digital image when it was created. Usually digital cameras, iPhones, Smart phones, and other phones can store these kind of data in the captured image. Stored data can be varies from camera to camera. You have refer manufactures manuals to see what kind of data it can be stored in the image using the device. However there are some common data that stored in each and every image while they were capturing. These Meta data are stored in the headers of the images files such as jpg, jpeg, png, etc. You can use these Meta data to identify images. -eta data are like DNA's of a digital image. They knows everything of the image.
Image Meta data contains following information in its headers.
- Date of Captured.
- Image Dimensions (width and height in pixels).
- Horizontal and Vertical Resolution of the image (96 dpi).
- Bit Depth (24).
- Color Representation (e.g. sRGB).
- Camera Model. (e.g. iPhone 4s)
- Camera Maker (e.g. Apple)
- Exposure Time (e.g. 1/15 sec.)
- F-stop (f/2.4)
- ISO Speed (ISO-640)
- Focal Length (4mm)
- EXIF Version (0221)
- Longitude (7;52;45)
- Latitude (6;48;21.454)
- Altitude (21)
- Item Type (in images jpg or png)
- File Size
- Owner.
Above Metadata are common in most images. But there are lot of other information that can embedded in the digital images. Those information are differ from device to device. So there can be dozens of individual Meta data properties in the captured image. You can read those information using PHP exif library.
Importance of image Metadata.
You can use image Metadata to identify various information about the media; such as the author of the image, when it was taken, where it taken from, what is the camera model, copyright information, etc. Copyright information are important for the ownership. GPS data (latitude, longitude, and altitude) are useful to find the location of the photo. GPS data also important to SEO purposes. They are help to search engines to find the relevant images. Image dimensions, bit depth, resolution, image size, image type are useful when dealing with images. The applications that use to edit images such as Photoshop, CorelDraw reads these Meta data to identify the the image.
What is Exif?
Exif stands for Exchangeable image file format. It is maintained by Camera and Imaging Products Association (CIPA). And published by Japan Electronics and Information Technology Industries Association (JEITA). Exif is a standard that describes the formats for images and tags used by digital cameras. It also contains the technical information about the captured image, Exif data are stored in image headers and does not directly visible to the viewer. You have to use special methods to manipulate and read exif data.
How to read Exif data using PHP?
PHP has a extension to deal with image files. it has functions to read Metadata of the image. Before you read the exif data through the PHP exif library, you have to do some configuration to your PHP installation.
Step 1: Enable php_mbstring and php_exif extensions in your php.ini file (PHP configuration file) To do this open your php.ini file and remove the semicolon in font of the extension.
Step 2: Restart Apache web server.
You have to keep in mind the order of the mbstring and exif libraries in the php.ini file. mbstring extension must be loaded before the php_exif extension. Otherwise you may get errors like follows.
"Fatal error: Call to undefined function exif_imagetype() in D:\Projects\read-image-meta-data\exif.php on line 3" -> Solution: enable php_mbstring.dll and php_exif.dll extension respectively in the php.ini file.
Now you are ready to read image Meta data using PHP exif library. Following examples show how to do it.
1. How to get the image type in PHP?
You can use exif_imagetype() function to determine the type of the image. It returns a integer value corresponding to the image type. If it fails to determine the image type it returns FALSE. Common image type constants are IMAGETYPE_JPEG (2), IMAGETYPE_PNG (3), IMAGETYPE_GIF(1), and IMAGETYPE_BMP (6). Likewise there are many other image file type constants defined in PHP. Please refer the manual. Instead of using image constants, you can also use relevant integer value for the switch cases. Following example shows you how to determine image type by reading exif data in PHP.
<?php
$image_file = 'D:\Photoes\2013\IMG_0213.jpg';
$image_type='';
if(file_exists($image_file)){
$type = exif_imagetype($image_file);
switch($type){
case IMAGETYPE_GIF:{
$image_type = 'GIF';
}break;
case IMAGETYPE_JPEG:{
$image_type = 'JPEG';
}break;
case IMAGETYPE_PNG:{
$image_type = 'PNG';
}break;
case IMAGETYPE_BMP:{
$image_type = 'BMP';
}break;
default:{
$image_type = 'Not gif,jpeg,png,or bmp';
};
}
echo 'Image type is '.$image_type;
}else{
echo 'File does not exists';
}
?>
2. How to read exif image headers using php?
exif_read_data() function reads the exif headers of a image file. This method returns an array of Meta data related to the image. Return details can be differ from camera to camera. Returned array is an associative array with header names as indexes. If no data found it returns FALSE. Following example prints all exif information of a given image.
<?php
$image_file = 'D:\Photoes\2012\DSC02710.JPG';
$image_type='';
$tab=str_repeat(' ',12);
if(file_exists($image_file)){
$details=exif_read_data($image_file);
foreach($details as $key=>$val){
echo $key.' = '.$val.'<br/>';
if(is_array($val)){
foreach($val as $key2=>$val2){
echo "<span style=\"margin-left:30px;\">".$key2.' = '.$val2.'</span><br/>';
}
}
}
}else{
echo 'File does not exists';
}
?>
3. Read image Meta data information more..
Sometimes you may be need to get the date that the photo was taken, Camera Model and Make, ISO Speed Ratings, GPS data, file size, resolution, width and height, and so on. Please refer following sample codes/scripts to read image Meta data. Some of these may not work with older images. But now most digital cameras embedded more image details with the produced image. So it is good practice to check your code with images which are taken from different camera models.
<?php
$image_file = 'D:\Photoes\2011\19062011433.jpg';
if(file_exists($image_file)){
$details = exif_read_data($image_file);
}else{
die('File does not exists');
}
?>
Please note that I have use above $details array for following examples. So you have to define it at the top of your script.
(a). How to get file name and file size using exif data?
echo 'File name is '.$details['FileName'].' and size is '.number_format(($details['FileSize']/1024),2).' Kb';
(b). How to get image create date time using php?
echo $details['DateTimeOriginal'];
(c). How to get camera model and make in php?
echo 'Make is '.$details['Make'].' and model is '.$details['Model'];
(d).How to get mime type using exif image data?
echo 'Mime type is '.$details['MimeType'];
(e). How to find image width and height using php exif details?
echo 'Height = '.$details['COMPUTED']['Height'].'px and Width = '.$details['COMPUTED']['Width'].'px';
or you can use following code.
echo 'Width = '.$details['ExifImageWidth'].'px Height = '.$details['ExifImageLength'];
4. How to read GPS data of an image taken from a digital camera?
GPS data helps to identify the where the image is taken from. It is useful load image location in a Google Map. You can use these latitude and longitude to create a Google Map. Most of latest digital cameras,smart phone cameras, and mobile phone cameras embedded the GPS data in to images taken. Please refer following examples. Before you read exif GPS data, please make sure your camera supports for automatic location tagging (geotagging) of images and videos. In the exif data array there is a key named 'SectionsFound'. First of all you have to check GPS section is included in it. If GPS section exists you can make sure that photo contains GPS data such as latitude and longitude.
<?php
$image_file = 'D:\Photoes\2011\IMG_0712.jpg';
if(file_exists($image_file)){
$details = exif_read_data($image_file);
$sections = explode(',',$details['SectionsFound']);
if(in_array('GPS',array_flip($sections))){
echo format_gps_data($details['GPSLatitude'],$details['GPSLatitudeRef']);
echo '<br/>';
echo format_gps_data($details['GPSLongitude'],$details['GPSLongitudeRef']);
}else{
die('GPS data not found');
}
}else{
die('File does not exists');
}
function format_gps_data($gpsdata,$lat_lon_ref){
$gps_info = array();
foreach($gpsdata as $gps){
list($j , $k) = explode('/', $gps);
array_push($gps_info,$j/$k);
}
$coordination = $gps_info[0] + ($gps_info[1]/60.00) + ($gps_info[2]/3600.00);
return (($lat_lon_ref == "S" || $lat_lon_ref == "W" ) ? '-'.$coordination : $coordination).' '.$lat_lon_ref;
}
?>