How to check character type in PHP?
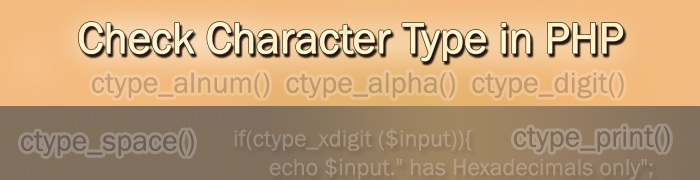
Check character types
When you are doing programming you may need to check the character types before process the data. For an example you have a web form that allows user to enter numbers only. So your program should accept numbers only. To perform this you have to check user input has valid numbers. Sometimes you may need to enter alpha numeric characters only. PHP has nice function set for checking character type. Using those methods you can check whether a character or string falls into a certain character class.
Note: These methods return TRUE if every character in the string matches the requested criteria otherwise FALSE.
Check alphanumeric characters in PHP.
You can check if all of the characters in the provided string containing alphanumeric (letters and integers) only by using ctype_alnum() function. This will return TRUE only if the provided string containing [A-Z a-z 0-9] characters. It does not matter simple or capital letters. You can use this method to validate user inputs such as user name, product name or product code, etc. See bellow example how to check if a string containing English alphabetic characters and numbers only. Remember sometimes you may be separate two words using a space character. This will cause to return FALSE value because "space" it not a valid alphanumeric character.
<?php $input_texts = array("Hello World","PHP","What?","2013"); foreach($input_texts as $input){ if(ctype_alnum($input)){ echo $input." has letters and numbers only<br/>"; }else{ echo $input." has not only letters and numbers<br/>"; } } ?>
Check Alphabetic letters in PHP.
Sometimes you may need to check a given string or text contains only letters (A-Z,a-z). In such cases you can use ctype_alpha() function to check if the string has only letters. This is useful when you are validating user name, first name or last name.
<?php $input_texts = array("lettersOnly","HAL9000","pami@gmail.com","PHP"); foreach($input_texts as $input){ if(ctype_alpha ($input)){ echo "Your input contains Letters only<br/>"; }else{ echo "Your input does not consist of all letters<br/>"; } } ?>
Check numeric characters in PHP.
Suppose that you have to check particular text or string contains only numbers 0-9. You can use ctype_digit() function to do that. This function will return TRUE only if the given string or text contains numbers 0 to 9 otherwise it returns FALSE. You can use this method to validate age of a person, passengers in a bus, members of a family. Because this will check integers only.
<?php $input_texts = array("2014","12.45","2011/11/11","No28","2nd"); foreach($input_texts as $input){ if(ctype_digit ($input)){ echo "Integers only<br/>"; }else{ echo "Not contain integers only, there are other characters too<br/>"; } } ?>
Check hexadecimal digits in PHP.
Suppose that you have to check particular text or string contains only hexadecimal digits. For an example to validate HTML color code you have to check all characters are valid hexadecimal digits. You can use ctype_xdigit() function to check this. Hexadecimal numbers contains letters from 'A' to 'F' and numbers from 0 to 9.
<?php $input_texts = array("2014","12.45","ABCDEF","ABXYZ","fffEEE","00EF",0.56); foreach($input_texts as $input){ if(ctype_xdigit ($input)){ echo $input." has Hexadecimals only"; }else{ echo $input." has not Hexadecimals only"; } } ?>
Check UPPER case and lower case in PHP.
Sometimes you may have to check the case sensitivity of a given word. Suppose that you have check captcha code in PHP, some websites allows you to enter captcha code without considering lower or upper case. If you need to check the case of a word or string in php you can use ctype_lower() function to determine lower case characters and ctype_upper() function to determine upper case characters.
<?php $input_texts = array("siple","hello world","CAPITAL","ProGAMMinG"); foreach($input_texts as $input){ if(ctype_upper($input)){ echo "uppercase character only"; }elseif(ctype_lower($input)){ echo "lowercase character"; }else{ echo "not only uppercase or lowercase"; } } ?>
Check whitespace characters/spaces in PHP.
You can check for white space characters using ctype_space() function. It returns TRUE only if all characters in the given text contains white spaces.
<?php $input_texts = array("text_a"=>" ", "text_b"=>"\n", "text_c"=>PHP_EOL, "text_d"=>" ", "text_e"=>"\r", "text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>"on the way"); foreach($input_texts as $key=>$input){ if(ctype_space($input)){ echo $key." space character only"; }else{ echo $key." not only spaces "; } } ?>Note: line feed(\n), vertical tab(\t), carriage return(\r) are considered as white spaces. In above example, all values except 'text_h' contains space characters.
Check control characters in PHP.
Control characters are also considered as non-printing characters. It is a code/set of characters that does not represent a written symbol. Using ctype_cntrl() function you can identify control characters. If a given text containing all characters of control it returns TRUE otherwise FALSE.
<?php $input_texts = array("text_a"=>"\f","text_b"=>"\n","text_c"=>PHP_EOL, "text_d"=>"\0","text_e"=>"\r","text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>"on the way","text_i"=>" ", "text_j"=>"\b"); foreach($input_texts as $key=>$input){ if(ctype_cntrl ($input)){ echo $key." is control character"; }else{ echo $key." is not a control character"; } } ?>Output: In above example values up to 'text_g' are contains control characters. others are not control characters.
Check printable characters in PHP.
Printable characters means characters that actually create output (including spaces/blanks). You can identify printable characters using ctype_print() function. Also you can determine all printable characters except white spaces using ctype_graph() function.
<?php $input_texts = array("text_a"=>"\f","text_b"=>"\n","text_c"=>PHP_EOL, "text_d"=>"\0","text_e"=>"\r","text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>" ","text_i"=>"welcome"); foreach($input_texts as $key=>$input){ if(ctype_print ($input)){ echo $key." has all printable character"; }else{ echo $key." is containing non printable character"; } } ?>Output: if you check using ctype_print() function , values all up to 'text_g' contains non printable characters. 'text_h ' and 'text_i' contains all printable characters. If you use ctype_graph() function, only 'text_i' considered as printable characters. Because space/white spaces are ignored.