How to validate drop down list using jQuery?
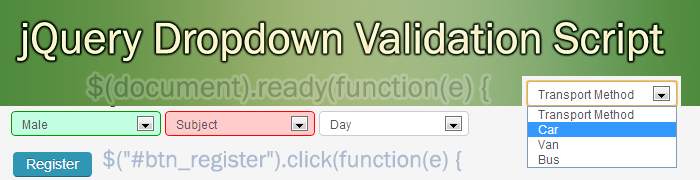
jQuery list menu/drop down/list box validation script
jQuery is the most easy way to do JavaScript client side validations. jQuery is fast and simple to use. You can reduce most of the code lines by using jQuery. However as a beginner it is better to learn drop down validation using pure JavaScript also. Drop down menu's or list boxes are very common is out day to day programming life. List menu's are use to get user inputs from list of given options. There can be single select or multiple select list boxes. This example based on a single select dropdown menu. First of all you have to download jQuery library. You can develop attractive web sites using jQuery and jQuery Color plugin. Always try to give meaningful and eye caching message to the user. Because UI development is an important factor.
Demo: Validate list menu using jQuery. Copy and paste given sample code into a blank HTML file and see how it works.Example 1: validate user's birthday.
This example use jQuery for validate user birthday. There are three dropdown lists for year, month, and day respectively. Before user click on submit button, he/she need to select his/her birthday correctly. You have to consider particular year is a leap year or not. If so, February month of that year has 29 days. Rest of years; it has 28 days for month of February. Then you have to consider maximum days for a month. If a user entered invalid date he will be informed to re-enter correct date. This example use JavaScript alert boxes for user notifications.
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(e) {
$("#btn_submit").click(function(e) {
var days_of_month=Array(31,29,31,30,31,30,31,31,30,31,30,31);
var year = parseInt($("#year").val());
if(year==0){
alert("Please select Year");
$("#year").focus();
return false;
}
var month = $("#month").val();
if(month==0){
alert("Please select Month");
$("#month").focus();
return false;
}
var day = $("#day").val();
if(day==0){
alert("Please select Day");
$("#day").focus();
return false;
}
if(month==2 && year%4==0 && day>days_of_month[1]){
alert("February can't have more than 29 days except in leap year");
$("#day").focus();
return false;
}else if(month==2 && day>28){
alert("February can't have more than 29 days except in leap year");
$("#day").focus();
return false;
}else if(day>days_of_month[month-1]){
alert($("#month :selected").text()+ " can't have more than "+days_of_month[month-1]+" days");
$("#day").focus();
return false;
}
alert("OK, Your selection is valid");
return true;
});
});
</script>
<title>Untitled Document</title>
</head>
<body>
<form action="http://www.latestcode.net" method="post" enctype="multipart/form-data" name="form_bd"><table border="0">
<tr>
<td>Select Your Birthday</td>
<td><label for="month">
<select name="year" id="year">
<option value="0" selected="selected">Year</option>
<option value="1980">1980</option>
<option value="1981">1981</option>
<option value="1982">1982</option>
<option value="1983">1983</option>
</select>
</label></td>
<td><select name="month" id="month" >
<option value="0" selected="selected">Month</option>
<option value="1">January</option>
<option value="2">February</option>
<option value="3">March</option>
<option value="4">April</option>
<option value="5">May</option>
<option value="6">June</option>
<option value="7">July</option>
<option value="8">August</option>
<option value="9">September</option>
<option value="10">October</option>
<option value="11">November</option>
<option value="12">December</option>
</select></td>
<td><label for="day"></label>
<select name="day" id="day" >
<option value="0">Day</option>
<option value="1">1</option><option value="2">2</option><option value="3">3</option><option value="4">4</option><option value="5">5</option><option value="6">6</option><option value="7">7</option><option value="8">8</option><option value="9">9</option><option value="10">10</option><option value="11">11</option><option value="12">12</option><option value="13">13</option><option value="14">14</option><option value="15">15</option><option value="16">16</option><option value="17">17</option><option value="18">18</option><option value="19">19</option><option value="20">20</option><option value="21">21</option><option value="22">22</option><option value="23">23</option><option value="24">24</option><option value="25">25</option><option value="26">26</option><option value="27">27</option><option value="28">28</option><option value="29">29</option><option value="30">30</option><option value="31">31</option>
</select></td>
</tr>
<tr>
<td> </td>
<td><input type="submit" name="btn_submit" id="btn_submit" value="Submit" /></td>
<td> </td>
<td> </td>
</tr>
</table>
</form>
</body>
</html>
Example 2: Validate dropdwon list using jQuery.
Suppose that you have to develop an online application form for student registration. You have to take subject, class day and gender of the student. Also you have to validate user input before he submit the form. Following example example how to validate above form in jQuery. This example use javascript alerts and change the background color of the list box for user notification. In your program, use one of them.
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<style type="text/css">
.selection_error{
border: 1px solid #F00;
background-color:#FFCCCC;
}
.correct_selection{
border: 1px solid #090;
background-color:#C1FFE0;
}
</style>
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(e) {
$.fn.changeBackground=function(component,message){
var status = (component.val()==0)?false:true;
if(status){//success
component.removeClass('selection_error');
component.addClass('correct_selection');
return true;
}else{//error
component.removeClass('correct_selection');
component.addClass('selection_error');
alert(message);
return false;
}
}
$("#btn_register").click(function(e) {
if(!$.fn.changeBackground($("#gender"),'Please select Gender')){
return false;
}
if(!$.fn.changeBackground($("#subject"),'Please select a Subject')){
return false;
}
if(!$.fn.changeBackground($("#class_day"),'Please select a Class Day')){
return false;
}
alert("OK selection is valid");
return true;
});
});
</script>
<title>Untitled Document</title>
</head>
<body>
<form id="form1" name="form1" method="post" action="http://www.latestcode.net"><table border="0">
<tr>
<td colspan="7">Online course registration</td>
</tr>
<tr>
<td> </td>
<td>
<label for="subject">
<select name="gender" id="gender">
<option value="0">Gender</option>
<option value="1">Male</option>
<option value="2">Female</option>
</select>
</label></td>
<td><label for="class_day">
<select name="subject" id="subject">
<option value="0" selected="selected">Subject</option>
<option value="1">Maths</option>
<option value="2">Physics</option>
<option value="3">Chemistry</option>
<option value="4">Biology</option>
<option value="5">Electronics</option>
</select>
</label></td>
<td><select name="class_day" id="class_day" >
<option value="0" selected="selected">Day</option>
<option value="1">Sunday</option>
<option value="2">Monday</option>
<option value="3">Tuesday</option>
<option value="4">Wednesday</option>
<option value="5">Thursday</option>
<option value="6">Friday</option>
<option value="7">Saturday</option>
</select></td>
</tr>
<tr>
<td> </td>
<td><input name="btn_register" type="submit" id="btn_register" value="Register" /></td>
<td> </td>
<td> </td>
</tr>
</table>
</form>
</body>
</html>
Example 3: Hotel booking system jQuery validation script
Suppose that you have to develop a hotel booking system. You have to take number of nights and number of heads (both adults and children), no of rooms as user inputs. All inputs should get through list menus / drop down boxes. And you have to validate all inputs before user submit the form.
This example based on jQuery Color plugin. So you have to download jQuery color plugin from Github.
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script src="//code.jquery.com/color/jquery.color-2.1.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function(e) {
$.fn.displayNotification=function(drop_down_element){
var status = drop_down_element.val();
var bg_color = (status==-1)?"#FF0000":"#009900";
drop_down_element.animate({
backgroundColor: ($).Color(bg_color).transition("transparent", 0.2)
},1000);
return (status==-1)?false:true;
}
$("#btn_book_now").click(function(e) {
if(!$.fn.displayNotification($('#no_of_nights'))){
return false;
}
if(!$.fn.displayNotification($('#adults'))){
return false;
}
if(!$.fn.displayNotification($('#children'))){
return false;
}
if(!$.fn.displayNotification($('#rooms'))){
return false;
}
return true;
});
});
</script>
<title>Untitled Document</title>
</head>
<body>
<form id="form3" name="form3" method="post" action="http://www.latestcode.net"><table border="0">
<tr>
<td colspan="8">Hotel Booking Form</td>
</tr>
<tr>
<td width="150">No of Nights</td>
<td width="200"><label for="no_of_nights"></label>
<select name="no_of_nights" id="no_of_nights">
<option value="-1">Nights</option>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
<option value="6">6</option>
<option value="7">7</option>
<option value="8">8</option>
<option value="9">9</option>
<option value="10">10</option>
</select></td>
<td width="200"> </td>
<td> </td>
</tr>
<tr>
<td>No of heads</td>
<td><label for="adults"></label>
<select name="adults" id="adults">
<option value="-1">Adults</option>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
<option value="6">6</option>
<option value="7">7</option>
<option value="8">8</option>
<option value="9">9</option>
<option value="10">10</option>
</select></td>
<td><select name="children" id="children">
<option value="-1">Children</option>
<option value="0">0</option>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
</select></td>
<td><label for="children"></label></td>
</tr>
<tr>
<td>No of Rooms</td>
<td><label for="rooms"></label>
<select name="rooms" id="rooms">
<option value="-1">Rooms</option>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
</select></td>
<td> </td>
<td> </td>
</tr>
<tr>
<td> </td>
<td><input name="btn_book_now" type="submit" id="btn_book_now" value="Book Now" /></td>
<td> </td>
<td> </td>
</tr>
<tr>
<td colspan="4"></td>
</tr>
</table>
</form>
</body>
</html>
Example 4: Validate application form in jQuery
Suppose that you have to create online job application form. User have to select age group, programming language, programming type (desktop or web) and his experience. See how to validate above registration form using jQuery.
This example also use jQuery Color plugin.
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script src="//code.jquery.com/color/jquery.color-2.1.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function(e) {
$.fn.displayErrors=function(drop_down_element){
var status = drop_down_element.val();
var bg_color = (status==0)?"#FF0000":"#009900";
drop_down_element.animate({
borderColor: ($).Color(bg_color).transition("transparent", 0.2)
},800);
return (status==0)?0:1;
}
$("#btn_apply_now").click(function(e) {
if(!$.fn.displayErrors($('#age_group'))){
return false;
}
if(!$.fn.displayErrors($('#programming_language'))){
return false;
}
if(!$.fn.displayErrors($('#experience'))){
return false;
}
return true;
});
});
</script>
<title>Untitled Document</title>
</head>
<body>
<form id="form4" name="form4" method="post" action="http://www.latestcode.net"><table border="0">
<tr>
<td colspan="7">Online application form for programmers</td>
</tr>
<tr>
<td>Age group</td>
<td><label for="age_group"></label>
<select name="age_group" id="age_group">
<option value="0" selected="selected">Age Group</option>
<option value="1">18-24</option>
<option value="2">25-30</option>
<option value="3">31-35</option>
<option value="4">36-40</option>
<option value="5">Above 40</option>
</select></td> </tr>
<tr>
<td>Main programming language</td>
<td>
<select name="programming_language" id="programming_language">
<option value="0" selected="selected">Programming Language</option>
<option value="1">Java</option>
<option value="2">PHP</option>
<option value="3">C#</option>
<option value="4">Python</option>
<option value="5">Ruby</option>
<option value="6">VB.Net</option>
<option value="7">C++</option>
</select></td>
</tr>
<tr>
<td>Experience</td>
<td>
<select name="experience" id="experience">
<option value="0" selected="selected"s>Experience</option>
<option value="1">1 Year</option>
<option value="2">2 Years</option>
<option value="3">3 Years</option>
<option value="4">4 Years</option>
<option value="5">5 Years</option>
<option value="6">6 Years</option>
<option value="7">7 Years</option>
<option value="8">8 Years</option>
<option value="9">9 Years</option>
<option value="10">10 Years</option>
<option value="11">More than 10 years</option>
</select></td>
</tr>
<tr>
<td><input name="btn_apply_now" type="submit" id="btn_apply_now" value="Apply Now" /></td>
<td> </td>
</tr>
<tr>
<td colspan="2"> </td>
</tr>
</table>
</form>
</body>
</html>
Example 5: Validate list menu using jQuery.
This example shows you validate list menu at the event of "onChange". As a example, a user will be submit a form without entering correct values. In such case, after click on submit button he will be displayed an error message. After that he will be select correct option. Then you have to reset error message. This demo program use additional message block to display a message to the user depending on his selection.
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script src="//code.jquery.com/color/jquery.color-2.1.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function(e) {
$.fn.showAnimation=function(drop_down_element){
var status = drop_down_element.val();
var bg_color = (status==0)?"#FF0000":"#009900";
drop_down_element.animate({
backgroundColor: ($).Color(bg_color).transition("transparent", 0.2)
},1000);
if(status==0){
$("#note5").html('Please fill all required fields');
$("#note5").animate({
backgroundColor: ($).Color(bg_color).transition("transparent", 0.2),
color:($).Color("#FFFFFF").transition("transparent",0)
},1000);
}else{
$("#note5").html('');
$("#note5").css('background-color','#FFFFFF');
}
return (status==0)?false:true;
}
$("#trip_from").change(function(e){
$.fn.showAnimation($(this));
});
$("#trip_to").change(function(e){
$.fn.showAnimation($(this));
});
$("#transport_type").change(function(e){
$.fn.showAnimation($(this));
});
$("#btn_booknow").click(function(e) {
var drop_downs = Array('trip_from','trip_to','transport_type');
for(var x=0;x<drop_downs.length;x++){
if(!$.fn.showAnimation($("#"+drop_downs[x]))){
return false;
}
}
$("#note5").html('Okay. Your selection is valid');
$("#note5").animate({
backgroundColor: ($).Color('#009900').transition("transparent", 0.2),
color:($).Color("#FFFFFF").transition("transparent",0)
},1000);
return true;
});
});
</script>
<title>Untitled Document</title>
</head>
<body>
<form id="form5" name="form5" method="post" action="http://www.latestcode.net"><table border="0">
<tr>
<td colspan="7">Online course registration</td>
</tr>
<tr>
<td> </td>
<td>
<select name="trip_from" id="trip_from">
<option value="0" selected="selected">From</option>
<option value="1">City 1</option>
<option value="2">City 2</option>
<option value="3">City 3</option>
</select> </td>
<td>
<select name="trip_to" id="trip_to">
<option value="0" selected="selected">To</option>
<option value="1">City 1</option>
<option value="2">City 2</option>
<option value="3">City 3</option>
</select> </td>
<td></td>
<td><select name="transport_type" id="transport_type" class="blog_drop_down">
<option value="0" selected="selected">Transport Method</option>
<option value="1">Car</option>
<option value="2">Van</option>
<option value="3">Bus</option>
</select></td>
</tr>
<tr>
<td> </td>
<td><input name="btn_booknow" type="submit" id="btn_booknow" value="Book Now" /></td>
<td colspan="3"><span id="note5" style="padding:5px;border-radius: 4px;"></span></td>
</tr>
</table>
</form>
</body>
</html>