Sunday, November 29, 2009
Get screen resolution in JavaScript
Limit characters in textarea
How to limit the number of characters entered in a text box or text aria in JavaScript?
JavaScript Character Counter | ||
1.Enter description (50 characters only). | ||
Characters remaining | view code | |
2.Limit characters in a text area. | ||
Enter number of characters you want to limit. | ||
Enter your text here | ||
Characters remaining | view code |
Try it!
1. Example code for limit number of input characters in a text area using JavaScript.
JavaScript Code2. Limit user input text using JavaScript.
Sunday, November 22, 2009
Validate Decimal Number
How to validate decimal number using JavaScript?
Using following javascript you can check
whether a particular number is a valid decimal number of not.
1. Validate decimal number. | ||
Enter a Decimal Number | ||
view code | ||
2. How to validate a decimal number on key press event. | ||
Enter a number | view code | |
3. Use following code to validate a decimal number with specified decimal places. | ||
Number of Decimal Points | view code | |
Enter Decimal Number | ||
Enter a value and click on submit button to test whether input is a valid decimal number or not. If you enter an invalid decimal number JavaScript alerts you, you have entered an invalid number. Otherwise it alerts the input is a valid decimal number.
1. Example code for validate a decimal number in JavaScript.
JavaScript Code<!--
function validateDecimalNumber(){
var value = document.getElementById("textDecimalNumber").value;
var message = "Please enter a decimal value";
if(value!=""){
if(isNaN(value)==true){
alert(message);
return false;
}else{
alert(value+" is a valid decimal number");
return true;
}
}else{
alert(message);
return false;
}
return false;
}
-->
</script>
<html>
<head>
<title>How to Validate Decimal Number in JavaScript </title>
</head>
<body>
<table border="0">
<tr>
<td>Enter Decimal Number </td>
<td>
<input name="textDecimalNumber" type="text" id="textDecimalNumber" />
</td>
</tr>
<tr>
<td> </td>
<td>
<input name="btnDecimalNumberValidator" type="submit" id="btnDecimalNumberValidator" onclick="javascript:validateDecimalNumber();" value="Validate" />
</td>
</tr>
</table>
</body>
</html>
2. Example code for validate a decimal number on key press event in JavaScript.
JavaScript Code<!--
function validate(){
var value = document.getElementById("txtDecimal").value;
var message = "Please enter a decimal value";
if(isNaN(value)==true){
alert(message);
document.getElementById("txtDecimal").select();
return false;
}else{
return true;
}
}
-->
</script>
<html>
<head>
<title>How to Validate Decimal Number in KeyPress Event in JavaScript </title>
</head>
<body>
<table border="0">
<tr>
<td>Enter Decimal Number </td>
<td>
<input name="textDecimalNumber" type="text" id="textDecimalNumber" />
</td>
</tr>
<tr>
<td> </td>
<td>
<input name="txtDecimal" type="text" id="txtDecimal" onkeyup="javascript:validate();" />
</td>
</tr>
</table>
</body>
</html>
3. Custom Decimal number validation using JavaScript.
<!--
function showDecimalPointError(){
var message="Please enter an integer value";
alert(message);
document.getElementById("textDecimalPoints").value="2";
return false;
}
//------------------------------------------------------------------
function CheckDecimalPoints(){
var decimalPoints=document.getElementById("textDecimalPoints").value;
for(var x=0;x<decimalPoints.length;x++){
if(decimalPoints.charAt(x)=="."){
showDecimalPointError();
}
if(isNaN(decimalPoints.charAt(x))){
showDecimalPointError();
}
}
return true;
}
//------------------------------------------------------------------
function customValidation(){
var preferredDecimalPoints=document.getElementById("textDecimalPoints").value;
var decimalPointCount=0;
var decimalNumber = document.getElementById("txtCustomValidation").value;
var pointFound=false;
var decimalPlaces=0;
for(var i=0;i< decimalNumber.length ;i++){
var _char=decimalNumber.charAt(i);
if(pointFound==true){
decimalPlaces++;
}
if(_char=="."){
pointFound=true;
decimalPointCount++;
if(decimalPointCount>1){
break;
}
}else if(isNaN(_char)){
setError()
return false;
}
}
if(decimalPointCount!=1){
setError()
return false;
}else if(decimalPlaces!=preferredDecimalPoints){
setError()
return false;
}else if(isNaN(decimalNumber)){
setError()
}else{
alert(decimalNumber+" is a valid decimal number");
}
return true;
}
//------------------------------------------------------------------
function setError(){
var message="Please enter a valid decimal number";
alert(message);
document.getElementById("txtCustomValidation").select();
document.getElementById("txtCustomValidation").focus();
return false;
}
//-------------------------------------------------------------------->
</script>
<head>
<title>How to Validate Decimal Number in JavaScript </title>
</head>
<body><table border="0">
<tr>
<td>Number of Decimal Points </td>
<td><label>
<input name="textDecimalPoints" type="text" id="textDecimalPoints" size="5" value="2" onkeyup="javascript:CheckDecimalPoints();" " />
</label></td>
</tr>
<tr>
<td>Enter Decimal Number </td>
<td><label>
<input name="txtCustomValidation" type="text" id="txtCustomValidation" />
</label></td>
</tr>
<tr>
<td> </td>
<td><label>
<input name="btnCustomValidation" type="submit" id="btnCustomValidation" value="Validate" onclick="javascript:customValidation();" />
</label></td>
</tr>
</table></body>
</html>
Sunday, November 15, 2009
How to upload files in PHP
How to upload files in PHP?
Remember that what are the file types that allow user to upload. Never allow users to upload files containing scripts such as file with .php, .asp, .aspx, .js, etc. extensions. If a user upload such a file it will be a big security risk to your web site. So make sure what are the file types allow to upload. First you have to filter files by extension to avoid this security risk. Also you have to check the files that already exists in the server with the same name. Otherwise previous file will be overwrite from the new one.
Configuration settings associated with file upload in PHPThere are some configuration settings in the php.ini file associated with file uploading.
| |||||||||||||||||||||
$_FILES['my_file']['x'] is an associative array in php. It contains details of the uploaded file such as file name, file size and file type. First file is uploaded to the temporary directory in the server machine as a temporary file. such as "C:\temp PHP move_uploaded_file() uploaded function copied the uploaded temporary file to the save location. Temporary file is deleted after the upload. | |||||||||||||||||||||
|
1. Example code for upload files using PHP
HTML Code<head>
<title>File Upload in PHP </title>
</head>
<body>
<table border="0">
<tr>
<td width="94">Select File </td>
<td width="96"><input type="file" id="my_file" name="my_file" /></td>
</tr>
<tr>
<td> </td>
<td>
<input name="btn_upload_file" type="submit" id="btn_upload_file" value="Upload" />
</td>
</tr>
</body>
</html>
if($_FILES['my_file']['error']==0){
$file_name = $_FILES['my_file']['name'];
$file_type = $_FILES['my_file']['type'];
$file_size = $_FILES['my_file']['size']/1024;
$temp_path = $_FILES['my_file']['tmp_name'];
$file_save_path="uploaded_files/".$file_name;
$uploaded = move_uploaded_file($temp_path,$file_save_path);
if($uploaded==true){
print("The file ".$file_name." has been uploaded successfully");
print("<br/> File Type : ".$file_type);
print("<br/> File Size : ".$file_size. "Kb");
}else{
}
}else{
print("Error while uploading the file ".$_FILES['my_file']['error']);
}
?>
JavaScript Validation
How to create arrays in PHP
How to create arrays in PHP?
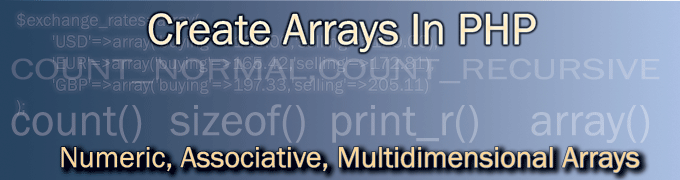
What is an array in php?
An Array is a collection of data values. It can also define as a collection of key/value pairs. An array can store multiple values in as a single variable. because array store values under a single variable name. Each array element has index which is used to access the array element. An array can also store diffrent type of data values. That means you can store integer and string values in the same array. PHP has lot of array functions to deal with arrays. You can store integers, strings, floats or objects in arrays. Arrays are very important in any programming language. You can get complete idea of arrays by reading this tutorial.
How to define an array in PHP?
$numbers = array(1,2,3,4,5,6,7,8,9,0);This will create an array of 10 elements. array is the pHP keyword that use to define a array. Not like other programming languages array size is not a fixed value. You can expand it at any time. That means you can store any value in the array you create. PHP will ready to adjust the size of the array.
Why arrays are important?
- Store similar data in a structured manner.
- Easy to access and change values.
- You can define any number of values (No need to define multiple variables).
- Save time and resources.
- To store temporary data.
In PHP there are two types of arrays.
- Numeric/Indexed Arrays
- Associative Arrays
Numeric Arrays
An indexed or a numeric array stores its all elements using numerical indexes. Normally indexed array begins with 0 index. When adding elements to the array index will be automatically increased by one. So always maximum index may be less than one to the size of the array. Following example shows you how to declare numeric arrays in pHPe.g. $programming_languages = array('PHP','Perl','C#');
Also you can define indexed/numeric arrays as follows.
$programming_languages[0]='PHP';
$programming_languages[1]='Perl';
$programming_languages[2]='C#';
Actually you don't need to specify the index, it will be automatically created. So you can also define it as bellow.
$programming_languages[]='PHP';
$programming_languages[]='Perl';
$programming_languages[]='C#';
Above array has three elements. The value 'PHP' has the index of 0 while 'C#' has index of 2. You can add new elements to this array. Then index will be increased one by one. That means the next element index will be 3. You can use that integer index to access array index as follows
echo $programming_languages[2];//This will be print 'C#'
or
echo $programming_languages{2}; //You can also use curly brackets
If you access an element which is not exists in the numeric array you will get an error message like Notice: Undefined offset: 4 in D:\Projects\array.php on line 7.
Associative Arrays
Associative arrays have keys and values. It is similar to two-column table. In associative arrays, key is use to access the its value. Every key in an associative array unique to that array. That means you can't have more than one value with the same key. Associative array is human readable than the indexed arrays. Because you can define a key for the specific element. You can use single or double quotes to define the array key. Following example explains you how to declare associative arrays in pHP
$user_info = array('name'=>'Nimal','age'=>20,'gender'=>'Male','status'=>'single');
Method 2:
$user_info['name']='Nimal';
$user_info['age']='20';
$user_info['gender']='Male';
$user_info['status']='Single'; Above array has four key pair values. That means array has 4 elements. Each element has a specific key. We use that key to access array element in associative arrays. You can't duplicate the array key. If there a duplicate key, the last element will be used.
echo $user_info['name'];//This will print Nimal
or
echo $user_info{'name'};
If you access an element which is not exists in the associative array you will get an error message like Notice: Undefined index: country in D:\Projects\array.php on line 11.
Multidimensional Arrays
Multidimensional arrays are arrays of arrays. That means one element of the array contains another array. Like wise you can define very complex arrays in pHP Suppose you have to store marks of the students according to their subjects in a class room using an array, You can use a multidimensional array to perform the task. See bellow example.Syntax:
$marks=array(
'nimal'=>array('English'=>67,'Science'=>80,'Maths'=>70),
'sanka'=>array('English'=>74,'Science'=>56,'Maths'=>60),
'dinesh'=>array('English'=>46,'Science'=>65,'Maths'=>52)
);
echo $marks['sanka']['English'];//This will print Sanka's marks for English
You can use array_keys() method to get all keys of an array. This will returns an array of keys.
print_r(array_keys($marks));
//This will output Array ( [0] => nimal [1] => sanka [2] => dinesh )
Note: you can use student number and subject number instead of using student name and subject name.
1. How to loop/iterate through an array in pHP?
if there are large number of elements in an array, It is not practical to access elements by manually. So you have to use loops for it. You can use foreach and for loops for access array elements. I think foreach loop is the most convenient method to access array elements. because you don't need to know the size of the array. In this tutorial you can learn iterate through arrays.
<?pHP
//Using foreach loop
$days = array('Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday');
foreach($days as $day){
echo $day.'<br/>';
}
//Using for loop
$programmingLanguages = array('PHP','Python','Perl','Java','C#','VB.Net','C++');
for($x=0;$x<count($programmingLanguages);$x++){
echo $programmingLanguages[$x].'<br/>';
}
//Also you can print all array elements using print_r() method. This is useful to determine array structure.
print_r($countries);
//This will out put Array ( [0] => SriLanka [1] => India [2] => America [3] => UK [4] => Japan [5] => China )
? >
2. How to get the size of an array using pHP?
Sometimes you may need to get how many elements exists in the array or the size of the array. You can use pHP in-built methods to find the size of a any array. See bellow examples. Also you can count all elements in a multidimensional array using count() function.
<?pHP
$countries = array('SriLanka','India','America','UK','Japan','China');
echo count($countries); //using count() method
echo '<br/>';
echo sizeof($countries);// using sizeof() method
//Get multidimensional array size
$exchange_rates=array(
'USD'=>array('buying'=>125.00,'selling'=>129.00),
'EUR'=>array('buying'=>165.42,'selling'=>172.81),
'GBP'=>array('buying'=>197.33,'selling'=>205.11)
);
echo count($exchange_rates,true);// this will out put 9
//or
echo count($exchange_rates,COUNT_RECURSIVE);
echo count($exchange_rates,COUNT_NORMAL);// this will out put 3
?>
Tuesday, November 10, 2009
Decimal Number Validation in javascript?
You can validate decimal number, floating point number using this javascript. If input number is not in the correct format it shows an error message.
Here is the javascript and the html code. Try to validate floating point number using this piece of code
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Untitled Document</title>
<style type="text/css">
.table_text{
font-family:Verdana, Arial, Helvetica, sans-serif;
font-size:11px;
}
</style>
<script type="text/javascript">
function decimal_check() {
var str=document.forms["decimal_form"].txtDecimalValue.value;
if(str!=""){
var regDeci=/^[-+]?\d+(\.\d+)?$/;
if(regDeci.test(str)==true){
alert(str+" Is Valid decimal number");
return true;
}else{
alert("Please enter valid number");
return false;
}
}else{
alert("Please enter valid number");
return false;
}
}
</script>
</head>
<body>
<form id="decimal_form" name="decimal_form" method="post" action="" onsubmit="return decimal_check();">
<table width="200" border="0" class="table_text">
<tr>
<td>Decimal Value </td>
<td><label>
<input name="txtDecimalValue" type="text" id="txtDecimalValue" />
</label></td>
<td> </td>
</tr>
<tr>
<td> </td>
<td><label>
<input name="btn_submit" type="submit" id="btn_submit" value="Submit" />
</label></td>
<td> </td>
</tr>
<tr>
<td> </td>
<td> </td>
<td> </td>
</tr>
</table>
</form>
</body>
</html>