How to create text file in PHP?
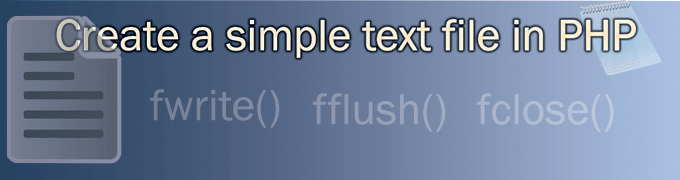
File handling is very important when doing programming. PHP has several methods to create text files. So you can make a text file in few steps. Before you creating files in your file system, you have to check whether the file exist or not. This prevents accidental file overwritten and data lost. Also you need have check enough permissions to write into the directory. You can simply use fopen() and fwrite() methods to create a file. It returns the number of bytes written to the file.
Demo - create text file in PHP?
1. Create simple text file in PHP?
Above demo shows a live example for creating a text file. Its code as follows. This code creates a new file with the given file name. fopen() method is use to create a file. fwrite() method use to write a string to the file. You can specify the length of the string that need to write in to the file. This is a optional parameter. If you didn't specify the length, it means total length of the string should be write to the file. Please note you have to give correct file name that support your platform. In this example I have explained how to add line brakes to the file.
Note: You can use PHP_EOL constant to make a line break. It automatically get the appropriate line break for the platform. Or you can use "\n" or "\r\n" for add line breaks to the file.
fflush() is use to forces a write of all buffered content to the file. This is useful when you are dumping large content in to a file.
<?php
$fileName="D:\projects\simpleFile.txt";
if(file_exists($fileName)==false){
$myFile = fopen($fileName, 'w');//w indicates you can write text to the file
$textToWrite = "Creating text file in php is very simple!";
$line2 =PHP_EOL."This is line two of your text file";
$line3 =PHP_EOL."You can use \"\\r\\n\" to break lines in your file";
fwrite($myFile,$textToWrite,strlen($textToWrite));
fflush($myFile);
fwrite($myFile,$line2);
fwrite($myFile,$line3);
fflush($myFile);
fclose($myFile);
}else{
print("File already exists. Please select another name");
}
? >
2. How to append text to a existing file in php?
Sometimes you may need to append some new data to an existing file. Following example shows how to add new text to file and modify it. This will append text, at the end of the file.
<?php
$fileName="D:\projects\existing-file.txt";
if(file_exists($fileName)){
$myFile = fopen($myFile, 'a+');
$new_text = PHP_EOL."This is a new content";
fwrite($myFile,$new_text);
fclose($myFile);
}else{
print("File does not exists");
}
?>
3. Useful functions when creating files in php?
Note: Before you use those methods you have to check if the file exists or not.
$fileName="D:\projects\existing-file.txt";
(a). How to get the file size?
echo "File size is ".number_format((filesize($fileName)/1024),2).' Kb';
(b). How to get the file created time in php?
date_default_timezone_set("Asia/Kolkata"); //First you have to specify the time zone
echo "File created time : " . date("Y M d H:i:s", filemtime($fileName));
echo "Last Modified: " . date("Y M d H:i:s", filectime($fileName));
(d). How to get the last accessed time of the file in php?
echo "Last Accessed: " . date("Y M d H:i:s", fileatime($fileName));
0 comments:
Post a Comment