How to generate random numbers using PHP?
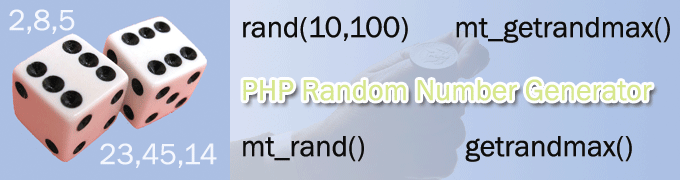
Random number generation methods
- Physical Methods
- Computational methods
Physical methods are earliest methods that used to generate random numbers. Coin flipping, dice throwing are examples for physical methods. Computational methods use mathematical algorithms to populate random numbers. Physical methods are very slow.
You can use rand(), or mt_rand() functions to generate random numbers in php
rand() function returns an integer value as a random number. Also you can specify lower and upper limit for generated number. also you can get the largest random number can be generated using rand() function. rand() function is 4 times slower than mt_rand(). So it is better to use mt_rand() method to make random numbers in php. Also mt_rand() produce bigger random value comparing to the rand() method. Usually it will be equal to maximum size of the integer value that can be produce by the system. maximum integer value is platform dependent.
Usage of random numbers
- Prepare online MCQ papers. (use to provide a random set of questions from a set of questions).
- Games (ex. dice, coin).
- Show selected posts (e.g. display Random posts in your website).
- Shuffle a play list.
- Generate CAPTCHA verification images.
- Create random passwords.
- Prevent caching web pages. (e.g. When using ajax calls append a random number at the end of the URL)
1. How to generate random numbers using php?
<?php
echo rand();//traditional pseudo-random number
echo '<br/>';
echo mt_rand();//Generate better random number. This is the newest method
?>
You can use above sample code to generate random integers in php. rand() function produce a small value than mt_rand(). All generated random numbers in the range of 0 to max random number that can be generated by the system.
2. How to determine maximum random values that can be generated using php?
<?php
echo getrandmax(); /* Maximum random value that can be generate using rand() method
This will out put 32767 in windows 7 - 32 bit computer */
echo '<br/>';
echo mt_getrandmax(); /* Maximum random value that can be generated using mt_rand() method This will out put 2147483647 in windows 7 - 32 bit machine */
?>
Maximum random number that can be generated by the system is platform dependent. It returns the largest possible integer value that can be return by rand() or mt_rand() function. Usually this is equal to the maximum integer value of your system. This also platform dependant. You can find the maximum integer value as follows.
<?phpecho PHP_INT_MAX;//maximum possible integer value
?>
3. How to generate a random number within a specific range in php?
<?php
$min = 10; // The lowest value of the random number to generate
$max = 100; // The maximum value to generate
echo rand($min,$max); /* Generate random number between 10 and 100*/
echo '<br/>';
echo mt_rand($min,$max); /* Get random value between 10 and 100 */
?>
Suppose that you need to generate random numbers between specific range, you can do it by passing minimum and maximum values to the rand() or mt_rand() functions. Then the generated random integers will be in the range that you have provide. By default minimum random number is 0 and the maximum random number is getrandmax() or mt_getrandmax() value. Maximum random numbers are platform dependent.
4. PHP random number examples
Think that you have to generate 10 random numbers between 1 and 100. Also you have to count the number of times each number generated. Also you need to print the summary of each occurrence Following example describes you how to do it.
<?php
$random_numbers =array();
for($x=0;$x<10;$x++){
$rnd = mt_rand(1,100);
if(array_key_exists($rnd,$random_numbers)){
$random_numbers[$rnd]++;
}else{
$random_numbers[$rnd]=1;
}
}
foreach($random_numbers as $number=>$times){
echo 'Number '.$number.' was generated '.$times.' times(s)<br/>';
}
?>
Random numbers are very useful. As a example you have a play list with 20 songs. What you have to do is play randomly selected songs of re arrange/shuffle the play list. You can use random numbers to perform the task.
Following example shows you how to select a day of the week randomly using php.
<?php
$days=array('Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday');
echo $days[mt_rand(0,count($days)-1)];
?>
Bellow example describes you how to generate random numbers 1 to 100
<?php
echo mt_rand(1,100);
?>
3 comments:
I want you to help me out with a small script requiring the idea of random number generation. Database will be involved. I want to create a 2 step authentication script that uses email.
First, the script should receive visitor's email address on a page carrying email as the only field.
Secondly, the script generates a 5 digits random number and save to a database against the visitor's email, and also sends a mail carrying the 5 digits code to the email address and also display a message requesting the visitor to login with the code received in his email inbox.
Next, the visitor is returns to login to proceed to a secured page on success or return back to attempt login with the code.
What will it take you to give me this help? I actually wanted to create the same thing for short code sent as sms to mobile phone instead of email but couldn't find a company that could assist so i decided to use this. Reach me through bravemarshal@gmail.com
Thanks for share good site :
http://idnpokeronline.me
http://134.19.190.108/dadu-online/
poker online uang asli terpercaya
From Me Pulsa777 Deposit Pulsa Tanpa Potongan
=====================================================
Hallo Semua Blog Ini Sangat Bagus Sekali Untuk Dibaca Saya Sangat Mengapreasinya
Mari Bergabung disini
Post a Comment