How to check character type in PHP?
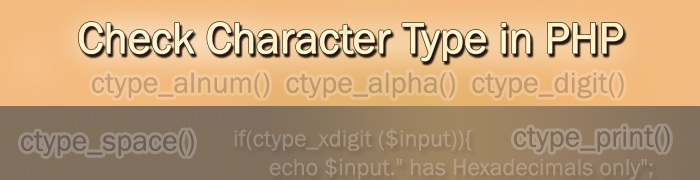
Check character types
When you are doing programming you may need to check the character types before process the data. For an example you have a web form that allows user to enter numbers only. So your program should accept numbers only. To perform this you have to check user input has valid numbers. Sometimes you may need to enter alpha numeric characters only. PHP has nice function set for checking character type. Using those methods you can check whether a character or string falls into a certain character class.
Note: These methods return TRUE if every character in the string matches the requested criteria otherwise FALSE.
Check alphanumeric characters in PHP.
You can check if all of the characters in the provided string containing alphanumeric (letters and integers) only by using ctype_alnum() function. This will return TRUE only if the provided string containing [A-Z a-z 0-9] characters. It does not matter simple or capital letters. You can use this method to validate user inputs such as user name, product name or product code, etc. See bellow example how to check if a string containing English alphabetic characters and numbers only. Remember sometimes you may be separate two words using a space character. This will cause to return FALSE value because "space" it not a valid alphanumeric character.
<?php $input_texts = array("Hello World","PHP","What?","2013"); foreach($input_texts as $input){ if(ctype_alnum($input)){ echo $input." has letters and numbers only<br/>"; }else{ echo $input." has not only letters and numbers<br/>"; } } ?>
Check Alphabetic letters in PHP.
Sometimes you may need to check a given string or text contains only letters (A-Z,a-z). In such cases you can use ctype_alpha() function to check if the string has only letters. This is useful when you are validating user name, first name or last name.
<?php $input_texts = array("lettersOnly","HAL9000","pami@gmail.com","PHP"); foreach($input_texts as $input){ if(ctype_alpha ($input)){ echo "Your input contains Letters only<br/>"; }else{ echo "Your input does not consist of all letters<br/>"; } } ?>
Check numeric characters in PHP.
Suppose that you have to check particular text or string contains only numbers 0-9. You can use ctype_digit() function to do that. This function will return TRUE only if the given string or text contains numbers 0 to 9 otherwise it returns FALSE. You can use this method to validate age of a person, passengers in a bus, members of a family. Because this will check integers only.
<?php $input_texts = array("2014","12.45","2011/11/11","No28","2nd"); foreach($input_texts as $input){ if(ctype_digit ($input)){ echo "Integers only<br/>"; }else{ echo "Not contain integers only, there are other characters too<br/>"; } } ?>
Check hexadecimal digits in PHP.
Suppose that you have to check particular text or string contains only hexadecimal digits. For an example to validate HTML color code you have to check all characters are valid hexadecimal digits. You can use ctype_xdigit() function to check this. Hexadecimal numbers contains letters from 'A' to 'F' and numbers from 0 to 9.
<?php $input_texts = array("2014","12.45","ABCDEF","ABXYZ","fffEEE","00EF",0.56); foreach($input_texts as $input){ if(ctype_xdigit ($input)){ echo $input." has Hexadecimals only"; }else{ echo $input." has not Hexadecimals only"; } } ?>
Check UPPER case and lower case in PHP.
Sometimes you may have to check the case sensitivity of a given word. Suppose that you have check captcha code in PHP, some websites allows you to enter captcha code without considering lower or upper case. If you need to check the case of a word or string in php you can use ctype_lower() function to determine lower case characters and ctype_upper() function to determine upper case characters.
<?php $input_texts = array("siple","hello world","CAPITAL","ProGAMMinG"); foreach($input_texts as $input){ if(ctype_upper($input)){ echo "uppercase character only"; }elseif(ctype_lower($input)){ echo "lowercase character"; }else{ echo "not only uppercase or lowercase"; } } ?>
Check whitespace characters/spaces in PHP.
You can check for white space characters using ctype_space() function. It returns TRUE only if all characters in the given text contains white spaces.
<?php $input_texts = array("text_a"=>" ", "text_b"=>"\n", "text_c"=>PHP_EOL, "text_d"=>" ", "text_e"=>"\r", "text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>"on the way"); foreach($input_texts as $key=>$input){ if(ctype_space($input)){ echo $key." space character only"; }else{ echo $key." not only spaces "; } } ?>Note: line feed(\n), vertical tab(\t), carriage return(\r) are considered as white spaces. In above example, all values except 'text_h' contains space characters.
Check control characters in PHP.
Control characters are also considered as non-printing characters. It is a code/set of characters that does not represent a written symbol. Using ctype_cntrl() function you can identify control characters. If a given text containing all characters of control it returns TRUE otherwise FALSE.
<?php $input_texts = array("text_a"=>"\f","text_b"=>"\n","text_c"=>PHP_EOL, "text_d"=>"\0","text_e"=>"\r","text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>"on the way","text_i"=>" ", "text_j"=>"\b"); foreach($input_texts as $key=>$input){ if(ctype_cntrl ($input)){ echo $key." is control character"; }else{ echo $key." is not a control character"; } } ?>Output: In above example values up to 'text_g' are contains control characters. others are not control characters.
Check printable characters in PHP.
Printable characters means characters that actually create output (including spaces/blanks). You can identify printable characters using ctype_print() function. Also you can determine all printable characters except white spaces using ctype_graph() function.
<?php $input_texts = array("text_a"=>"\f","text_b"=>"\n","text_c"=>PHP_EOL, "text_d"=>"\0","text_e"=>"\r","text_f"=>"\t", "text_g"=>"\n\r\t","text_h"=>" ","text_i"=>"welcome"); foreach($input_texts as $key=>$input){ if(ctype_print ($input)){ echo $key." has all printable character"; }else{ echo $key." is containing non printable character"; } } ?>Output: if you check using ctype_print() function , values all up to 'text_g' contains non printable characters. 'text_h ' and 'text_i' contains all printable characters. If you use ctype_graph() function, only 'text_i' considered as printable characters. Because space/white spaces are ignored.
8 comments:
that too good
Đến với nhanh như điện của chúng tôi bạn sẽ được sử dụng những dịch vụ vận chuyển với tốc độ có thể nói là nhanh như điện.
Các dịch vụ của chúng tôi như gửi hàng đi nha trang, chuyển hàng đi hà nội, dịch vụ vận chuyển gửi hàng vào sài gòn. Đặc biệt khi bạn cần gửi hàng từ sài gòn đi nha trang chúng tôi cũng sẵn sàng đáp ứng nhu cầu của bạn.
Ngoài ra khi cần mua hàng trên amazon hay vận chuyển hàng mỹ về việt nam thì cũng chỉ cần liên hệ với chúng tôi.
Các nhà khoa học Nhật Bản sẽ giúp bạn tìm ra phương cách tốt nhất để vừa tăng cường sức khỏe, vừa chống lại ung thư một cách an toàn và hiệu quả nhất. Cùng tìm hiểu công dụng của thuốc fucoidan hỗ trợ điều trị ung thư Fucoidan Umi No Shizuku giá bao nhiêu? Mua ở đâu?
Fucoidan Umi No Shizuku là thực phẩm chức năng hỗ trợ điều trị ung thư của Nhật Bản được đánh giá cao nhất hiện nay. Tham khảo cách dùng thuốc Fucoidan. Fucoidan là kết quả của nhiều năm nghiên cứu và tổng hợp của các bác sĩ hàng đầu tại Nhật. Đây là sản phẩm cao cấp từ hãng dược phẩm Kamerycah Inc. Fucoidan là thuốc gì ? một trong những thương hiệu tiên phong trong lĩnh vực ứng dụng thực phẩm biển phục vụ việc chữa bệnh để mang đến sức khỏe tối ưu nhất cho người sử dụng. cách sử dụng thuốc Fucoidan. Bài viết này sẽ giúp bạn tìm hiểu về sản phẩm thuốc hỗ trợ điều trị ung thư Fucoidan Umi No Shizuku giá bao nhiêu? Mua ở đâu?
Nốt ruồi trên mũi
Nếu bạn có một nốt ruồi trên mũi: bạn sẽ gặp được nhiều điều may mắn trong cuộc sống. Trong chuyện tình cảm, những người sở hữu nốt ruồi này thường được nhiều người thích và theo đuổi.
Nốt ruồi phụ nữ mọc ở đâu giàu http://giantinhmachchan.net/p/not-ruoi-phu-nu-moc-o-dau-thi-giau-sang.html
Tuy nhiên, họ lại không lý trí nhiều lắm trong chuyện kết hôn, do vậy mà dễ dẫn tới việc chọn sai người trăm năm. Còn khi đã kết hôn, họ có khả năng làm cho đời sống chăn gối của hai vợ chồng hoàn toàn viên mãn.
Nốt ruồi ở chính giữa mu bàn chân
Không ít người chỉ thích nghiên cứu về nốt ruồi ở lòng bàn chân mà quên đi tướng nốt ruồi ở mu bàn chân. Theo quan điểm nhân tướng học, người có nốt ruồi ở chính giữa mu bàn chân thường được hưởng cuộc sống phú quý.
Nuối ruồi ở ngón chân : http://giantinhmachchan.net/p/not-ruoi-o-ngon-chan-noi-len-tinh-cach-va-van-menh-nao.html
Nốt ruồi mọc trong tai
Phụ nữ có nốt ruồi mọc trong tai là những người vô cùng giàu có, tương lai tươi sáng, trường thọ, nhất là khi về già bạc vàng tiêu xả láng.
Tuy chỉ mới xuất hiện ở thị trường Việt Nam trong vài năm trở lại đây, nhưng sản phẩm http://trinutgotchan.com/p/thuoc-fucoidan-co-may-loai-fucoidan-loai-nao-tot.html đã nhanh chóng được nhiều người biết tới bởi khả năng kỳ diệu giúp đẩy lùi ung thư hiệu quả. http://thuocmatngu.com/cong-dung-cua-thuoc-fucoidan-tri-ung-thu-cua-my.html đang dần khẳng định vị trí của mình trong y học với khả năng chữa trị ung thư rất hiệu quả. http://muahangamazon.net/vi-sao-thuoc-fucoidan-doctors-best-best-duoc-ua-chuong-hien-nay.html nhiều bệnh nhân ung thư thử dùng Fucoidan và đã tìm thấy hi vọng cho mình. Hiện tại các loại sản phẩm chứa hoạt chất Fucoidan có mặt ở thị trường Việt Nam rất đa dạng về chủng loại và giá cả, http://thuốctrịsẹo.com/thuoc-fucoidan-ho-tro-tri-ung-thu-co-tot-khong-mua-o-dau.html tìm một địa chỉ tin cậy để mua sản phẩm Fucoidan là điều quan trọng không kém. tuy nhiên, hiện nay lại chưa có một loại phương pháp nào có thể điều trị tận gốc căn bệnh này. Ngoài ra, http://muahangtrenebay.com/tac-dung-cua-thuoc-fucoidan-gia-thuoc-fucoidan-hien-nay.html khác những phương pháp như xạ trị, sử dụng thuốc Tây y ảnh hưởng rất nhiều đến bệnh nhân do có nguồn gốc hóa học.
Hello, I enjoy reading all of your article. I wanted to write a little comment to support you. itunes store login
Thankyou for sharing :
http://134.19.190.107/
http://134.19.190.108/
This is an awesome post. Really very informative and creative contents. This concept is a good way to enhance knowledge. I like it and help me to development very well. Thank you for this brief explanation and very nice information. Well, got good knowledge.
Informatica training course
Informatica course
Informatica training
Post a Comment